Python
Python is a very simple yet very powerful object oriented programming language.
Python is developed by Guido van Rossum. Guido van
Rossum started implementing Python in 1989. Python is a very simple
programming language so even if you are new to programming, you can
learn python without facing any issues.
Interesting fact: Python is named after the comedy television show Monty Python’s Flying Circus. It is not named after the Python snake.
Features of Python programming language
1.Readable: Python is a very readable language.
2.Easy to Learn: Learning python is easy as this is
a expressive and high level programming language, which means it is
easy to understand the language and thus easy to learn.
3.Cross platform: Python is available and can run
on various operating systems such as Mac, Windows, Linux, Unix etc. This
makes it a cross platform and portable language.
4.Open Source: Python is a open source programming language.
5.Large standard library: Python comes with a large
standard library that has some handy codes and functions which we can
use while writing code in Python.
6.Free: Python is free to download and use. This means you can download it for free and use it in your application. .
Python is an example of a FLOSS (Free/Libre Open Source Software),
which means you can freely distribute copies of this software, read its
source code and modify it.
7.Supports exception handling: If you are new, you
may wonder what is an exception? An exception is an event that can occur
during program exception and can disrupt the normal flow of program.
Python supports exception handling which means we can write less error
prone code and can test various scenarios that can cause an exception
later on.
8.Advanced features: Supports generators and list comprehensions. We will cover these features later.
9.Automatic memory management: Python supports
automatic memory management which means the memory is cleared and freed
automatically. You do not have to bother clearing the memory.
What Can You Do with Python?
You may be wondering what all are the applications of Python. There
are so many applications of Python, here are some of the them.
1. Web development – Web framework like Django and Flask are based on Python. They help you write server side code which helps you manage database, write backend programming logic, mapping urls etc.
1. Web development – Web framework like Django and Flask are based on Python. They help you write server side code which helps you manage database, write backend programming logic, mapping urls etc.
2. Machine learning – There are many machine learning applications
written in Python. Machine learning is a way to write a logic so that a
machine can learn and solve a particular problem on its own. For
example, products recommendation in websites like Amazon, Flipkart, eBay
etc. is a machine learning algorithm that recognises user’s interest.
Face recognition and Voice recognition in your phone is another example
of machine learning.
3. Data Analysis – Data analysis and data visualisation in form of charts can also be developed using Python.
4. Scripting – Scripting is writing small programs to automate simple
tasks such as sending automated response emails etc. Such type of
applications can also be written in Python programming language.
5. Game development – You can develop games using Python.
6. You can develop Embedded applications in Python.
7. Desktop applications – You can develop desktop application in Python using library like TKinter or QT.
How to install Python
Python installation is pretty simple, you can install it on any operating system such as Windows, Mac OS X, Ubuntu etc. Just follow the steps.
You can install Python on any operating system such as Windows, Mac OS X, Linux/Unix and others.
To install the Python on your operating system, go to this link: https://www.python.org/downloads/. You will see a screen like this.
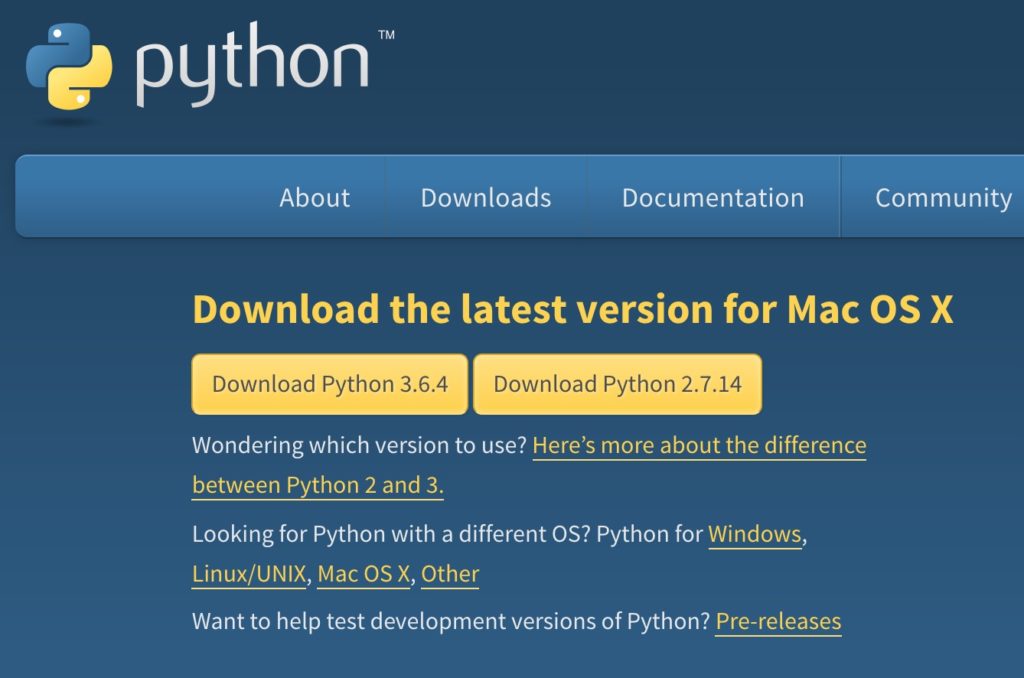
To install the Python on your operating system, go to this link: https://www.python.org/downloads/. You will see a screen like this.
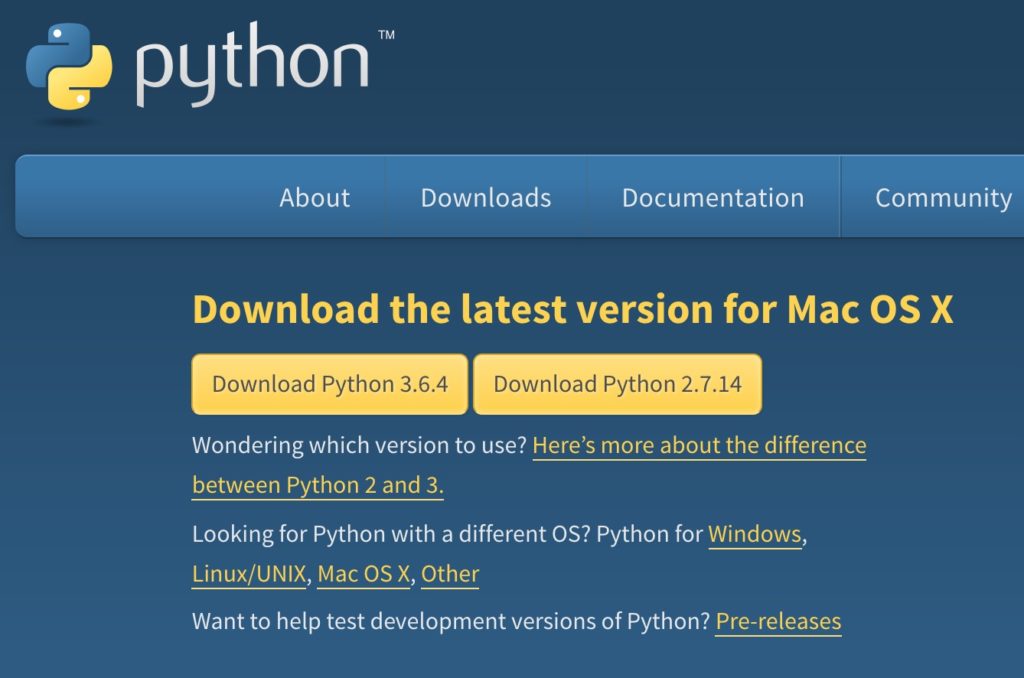
I would recommend you to download the latest version of Python 3
Lets see how a comment looks like in Python.
1. Single line comment
2. Multiple line comment
# This is just a comment. Anything written here is ignored by Python
''' This is a multi-line comment '''
''' We are writing a simple program here First print statement. This is a multiple line comment. '''
Comments in Python Programming
A comment is text that doesn’t affect the outcome of a code, it is just a piece of text to let someone know what you have done in a program or what is being done in a block of code. This is especially helpful when someone else has written a code and you are analysing it for bug fixing or making a change in logic, by reading a comment you can understand the purpose of code much faster then by just going through the actual code.Lets see how a comment looks like in Python.
# This is just a text, it won't be executed. print("Python comment example")
Types of Comments in Python
There are two types of comments in Python.1. Single line comment
2. Multiple line comment
Single line comment
In python we use # special character to start the comment. Lets take few examples to understand the usage.# This is just a comment. Anything written here is ignored by Python
Multi-line comment:
To have a multi-line comment in Python, we use triple single quotes at the beginning and at the end of the comment, as shown below.''' This is a multi-line comment '''
Python Comments Example
In this Python program we are seeing three types of comments. Single line comment, multi-line comment and the comment that is starting in the same line after the code.''' We are writing a simple program here First print statement. This is a multiple line comment. '''
print("Hello Guys")
# Second print statement
print("How are You all?")
print("Welcome to BeginnersBook") # Third print statement
# character inside quotes
When # character is encountered inside quotes, it is not considered as comment. For example:print("#this is not a comment")Output:
#this is not a comment
Python Variables with examples
Variables are used to store data, they take memory space based on the type of value we assigning to them. Creating variables in Python is simple, you just have write the variable name on the left side of = and the value on the right side, as shown below. You do not have to explicitly mention the type of the variable, python infer the type based on the value we are assigning.num = 100 #num is of type int str = "Chaitanya" #str is of type string
Variable name – Identifiers
Variable name is known as identifier. There are few rules that you have to follow while naming the variables in Python.1. The name of the variable must always start with either a letter or an underscore (_). For example: _str, str, num, _num are all valid name for the variables.
2. The name of the variable cannot start with a number. For example: 9num is not a valid variable name.
3. The name of the variable cannot have special characters such as %, $, # etc, they can only have alphanumeric characters and underscore (A to Z, a to z, 0-9 or _ ).
4. Variable name is case sensitive in Python which means
num
and NUM
are two different variables in python.Python Variable Example
num = 100
str = "BeginnersBook"
print(num)
print(str)
1. Immutable data types – Values cannot be changed.
2. Mutable data types – Values can be changed
Immutable data types in Python are:
1. Numbers
2. String
3. Tuple
Mutable data types in Python are:
1. List
2. Dictionaries
3. Sets
Variable name is known as identifier. There are few rules that you have to follow while naming the variables in Python.
For example here the variable is of integer type that holds the value 10. The name of the variable, which is
2. The name of the variable cannot start with a number. For example: 9num is not a valid variable name.
3. The name of the variable cannot have special characters such as %, $, # etc, they can only have alphanumeric characters and underscore (A to Z, a to z, 0-9 or _ ).
4. Variable name is case sensitive in Python which means
Immutable Data types in Python
1. Numeric
2. String
3. Tuple
Mutable Data types in Python
1. List
2. Dictionary
3. Set
Float – Values with decimal points are the float values, there is no need to specify the data type in Python. It is automatically inferred based on the value we are assigning to a variable. For example here fnum is a float data type.
# float number fnum = 34.45 print(fnum) print("Data Type of variable fnum is", type(fnum))
Python multiple assignment
We can assign multiple variables in a single statement like this in Python.x = y = z = 99 print(x) print(y) print(z)Output:
99 99 99Another example of multiple assignment
a, b, c = 5, 6, 7 print(a) print(b) print(c)
Plus and concatenation operation on the variables
x = 10 y = 20 print(x + y) p = "Hello" q = "World" print(p + " " + q)
Data Types
A data type defines the type of data, for example 123 is an integer data while “hello” is a String type of data. The data types in Python are divided in two categories:1. Immutable data types – Values cannot be changed.
2. Mutable data types – Values can be changed
Immutable data types in Python are:
1. Numbers
2. String
3. Tuple
Mutable data types in Python are:
1. List
2. Dictionaries
3. Sets
Python Keywords and Identifiers with examples
A python keyword is a reserved word which you can’t use as a name of
your variable, class, function etc. These keywords have a special
meaning and they are used for special purposes in Python programming
language. For example – Python keyword “while” is used for while loop
thus you can’t name a variable with the name “while” else it may cause
compilation error.
There are total 33 keywords in Python 3.6. To get the keywords list
on your operating system, open command prompt (terminal on Mac OS) and
type “Python” and hit enter. After that type help()
and hit enter. Type keywords
to get the list of the keywords for the current python version running on your operating system.Welcome to Python 3.6's help utility! ... help> keywords Here is a list of the Python keywords. Enter any keyword to get more help. False def if raise None del import return True elif in try and else is while as except lambda with assert finally nonlocal yield break for not class from or continue global pass
Example of Python keyword
In the following example we are using while keyword to create a loop for displaying the values of variables num as long as they are greater than 5.num = 10 while num>5: print(num) num -= 1
Python Identifiers
In the last article, we discussed about variables in Python.Variable name is known as identifier. There are few rules that you have to follow while naming the variables in Python.
For example here the variable is of integer type that holds the value 10. The name of the variable, which is
num
is called identifier.num = 101. The name of the variable must always start with either a letter or an underscore (_). For example: _str, str, num, _num are all valid name for the variables.
2. The name of the variable cannot start with a number. For example: 9num is not a valid variable name.
3. The name of the variable cannot have special characters such as %, $, # etc, they can only have alphanumeric characters and underscore (A to Z, a to z, 0-9 or _ ).
4. Variable name is case sensitive in Python which means
num
and NUM
are two different variables in python.Python identifier example
In the following example we have three variables. The name of the variablesnum
, _x
and a_b
are the identifiers.# few examples of identifiers num = 10 print(num) _x = 100 print(_x) a_b = 99 print(a_b)
Python Data Types
Data type defines the type of the variable, whether it is an integer variable, string variable, tuple, dictionary, list etc. In this guide, you will learn about the data types and their usage in Python.Python data types
Python data types are divided in two categories, mutable data types and immutable data types.Immutable Data types in Python
1. Numeric
2. String
3. Tuple
Mutable Data types in Python
1. List
2. Dictionary
3. Set
1. Numeric Data Type in Python
Integer – In Python 3, there is no upper bound on the integer number which means we can have the value as large as our system memory allows.# Integer number num = 100 print(num) print("Data Type of variable num is", type(num))Long – Long data type is deprecated in Python 3 because there is no need for it, since the integer has no upper limit, there is no point in having a data type that allows larger upper limit than integers.
Float – Values with decimal points are the float values, there is no need to specify the data type in Python. It is automatically inferred based on the value we are assigning to a variable. For example here fnum is a float data type.
# float number fnum = 34.45 print(fnum) print("Data Type of variable fnum is", type(fnum))
Complex Number – Numbers with real and imaginary
parts are known as complex numbers. Unlike other programming language
such as Java, Python is able to identify these complex numbers with the
values. In the following example when we print the type of the variable
cnum, it prints as complex number.
0b(zero + ‘b’) and 0B(zero + ‘B’) – Binary Number
0o(zero + ‘o’) and 0O(zero + ‘O’) – Octal Number
0x(zero + ‘x’) and 0X(zero + ‘X’) – Hexadecimal Number
# integer equivalent of binary number 101
# complex number cnum = 3 + 4j print(cnum) print("Data Type of variable cnum is", type(cnum))
Binary, Octal and Hexadecimal numbers
In Python we can print decimal equivalent of binary, octal and hexadecimal numbers using the prefixes.0b(zero + ‘b’) and 0B(zero + ‘B’) – Binary Number
0o(zero + ‘o’) and 0O(zero + ‘O’) – Octal Number
0x(zero + ‘x’) and 0X(zero + ‘X’) – Hexadecimal Number
# integer equivalent of binary number 101
num = 0b101
print(num)
# integer equivalent of Octal number 32
num2 = 0o32
print(num2)
# integer equivalent of Hexadecimal number FF
num3 = 0xFF
print(num3)
2. Python Data Type – String
String is a sequence of characters in Python. The data type of String in Python is called “str”.Strings in Python are either enclosed with single quotes or double quotes. In the following example we have demonstrated two strings one with the double quotes and other string s2 with the single quotes.
# Python program to print strings and type s = "This is a String" s2 = 'This is also a String' # displaying string s and its type print(s) print(type(s)) # displaying string s2 and its type print(s2) print(type(s2))
3. Python Data Type – Tuple
Tuple is immutable data type in Python which means it cannot be changed. It is an ordered collection of elements enclosed in round brackets and separated by commas.# tuple of integers
t1 = (1, 2, 3, 4, 5)
# prints entire tuple
print(t1)
# tuple of strings
t2 = ("hi", "hello", "bye")
# loop through tuple elements
for s in t2:
print (s)
# tuple of mixed type elements
t3 = (2, "Lucy", 45, "Steve")
'''
Print a specific element
indexes start with zero
'''
print(t3[2])
4. Python Data Type – List
List is similar to tuple, it is also an ordered collection of elements, however list is a mutable data type which means it can be changed unlike tuple which is an immutable data type.A list is enclosed with square brackets and elements are separated by commas.
# list of integers lis1 = (1, 2, 3, 4, 5) # prints entire list print(lis1) # list of strings lis2 = ("Apple", "Orange", "Banana") # loop through tuple elements for x in lis2: print (x) # List of mixed type elements lis3 = (20, "Chaitanya", 15, "BeginnersBook") ''' Print a specific element in list indexes start with zero ''' print("Element at index 3 is:",lis3[3])
5. Python Data Type – Dictionary
Dictionary is a collection of key and value pairs. A dictionary doesn’t allow duplicate keys but the values can be duplicate. It is an ordered, indexed and mutable collection of elements.The keys in a dictionary doesn’t necessarily to be a single data type, as you can see in the following example that we have 1 integer key and two string keys.
# Dictionary example dict = {1:"Chaitanya","lastname":"Singh", "age":31} # prints the value where key value is 1 print(dict[1]) # prints the value where key value is "lastname" print(dict["lastname"]) # prints the value where key value is "age" print(dict["age"])
6. Python Data Type – Set
A set is an unordered and unindexed collection of items. This means when we print the elements of a set they will appear in the random order and we cannot access the elements of set based on indexes because it is unindexed.Elements of set are separated by commas and enclosed in curly braces. Lets take an example to understand the sets in Python.
# Set Example myset = {"hi", 2, "bye", "Hello World"} # loop through set for a in myset: print(a) # checking whether 2 exists in myset print(2 in myset) # adding new element myset.add(99) print(myset)
Python If Statement explained with examples
if statements are control flow statements which helps us to run a
particular code only when a certain condition is satisfied. For example,
you want to print a message on the screen only when a condition is true
then you can use if statement to accomplish this in programming. In
this guide, we will learn how to use if statements in Python programming with the help of examples.
There are other control flow statements available in Python such as if..else, if..elif..else,
nested if etc. However in this guide, we will only cover the if statements, other control statements are covered in separate tutorials.
Syntax of If statement in Python
The syntax of if statement in Python is pretty simple.if condition: block_of_code
If statement flow diagram
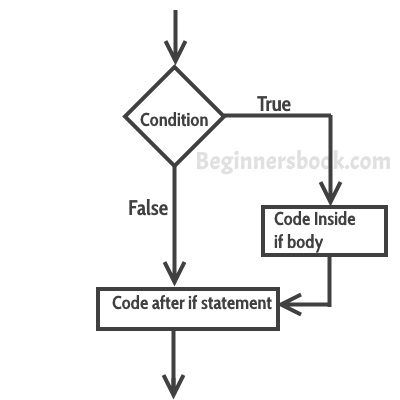
Python – If statement Example
flag = True if flag==True: print("Welcome") print("To") print("BeginnersBook.com")Output:
Welcome To BeginnersBook.comIn the above example we are checking the value of flag variable and if the value is True then we are executing few print statements. The important point to note here is that even if we do not compare the value of flag with the ‘True’ and simply put ‘flag’ in place of condition, the code would run just fine so the better way to write the above code would be:
flag = True if flag: print("Welcome") print("To") print("BeginnersBook.com")By seeing this we can understand how if statement works. The output of the condition would either be true or false. If the outcome of condition is true then the statements inside body of ‘if’ executes, however if the outcome of condition is false then the statements inside ‘if’ are skipped. Lets take another example to understand this:
flag = False if flag: print("You Guys") print("are") print("Awesome")The output of this code is none, it does not print anything because the outcome of condition is ‘false’.
Python if example without boolean variables
In the above examples, we have used the boolean variables in place of conditions. However we can use any variables in our conditions. For example:num = 100 if num < 200: print("num is less than 200")Output:
num is less than 200
Python If else Statement Example
We use if statements when we need to execute a certain block of Python code when a particular condition is true. If..else statements are like extension of ‘if’ statements, with the help of if..else we can execute certain statements if condition is true and a different set of statements if condition is false. For example, you want to print ‘even number’ if the number is even and ‘odd number’ if the number is not even, we can accomplish this with the help of if..else statement.Python – Syntax of if..else statement
if condition: block_of_code_1 else: block_of_code_2block_of_code_1: This would execute if the given condition is true
block_of_code_2: This would execute if the given condition is false
If..else flow control
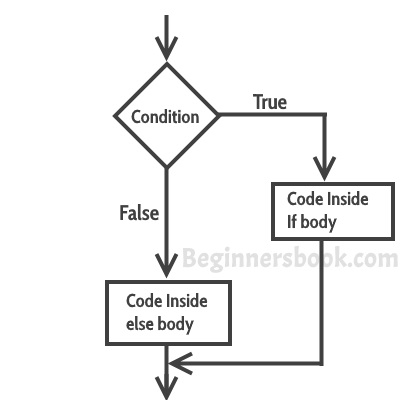
If-else example in Python
num = 22 if num % 2 == 0: print("Even Number") else: print("Odd Number")
Python If elif else statement example
we will learn if elif else statement in Python. The if..elif..else statement is used when we need to check multiple conditions.
Syntax of if elif else statement in Python
This way we are checking multiple conditions.if condition: block_of_code_1 elif condition_2: block_of_code_2 elif condition_3: block_of_code_3 .. .. .. else: block_of_code_nNotes:
1. There can be multiple ‘elif’ blocks, however there is only ‘else’ block is allowed.
2. Out of all these blocks only one block_of_code gets executed. If the condition is true then the code inside ‘if’ gets executed, if condition is false then the next condition(associated with elif) is evaluated and so on. If none of the conditions is true then the code inside ‘else’ gets executed.
Python – if..elif..else statement example
In this example, we are checking multiple conditions using if..elif..else statement.num = 1122 if 9 < num < 99: print("Two digit number") elif 99 < num < 999: print("Three digit number") elif 999 < num < 9999: print("Four digit number") else: print("number is <= 9 or >= 9999")
Python Nested If else statement
we will learn the nesting of these control statements.
When there is an if statement (or if..else or if..elif..else) is
present inside another if statement (or if..else or if..elif..else) then
this is calling the nesting of control statements.Nested if..else statement example
Here we have a if statement inside another if..else statement block. Nesting control statements makes us to check multiple conditions.num = -99 if num > 0: print("Positive Number") else: print("Negative Number") #nested if if -99<=num: print("Two digit Negative Number")Output:
Negative Number Two digit Negative Number
Python for Loop explained with examples
A loop is a used for iterating over a set of statements repeatedly. In Python we have three types of loops for, while and do-while. In this guide, we will learn for loop and the other two loops are covered in the separate tutorials.Syntax of For loop in Python
for <variable> in <sequence>: # body_of_loop that has set of statements # which requires repeated executionHere <variable> is a variable that is used for iterating over a <sequence>. On every iteration it takes the next value from <sequence> until the end of sequence is reached.
Lets take few examples of for loop to understand the usage.
Python – For loop example
The following example shows the use of for loop to iterate over a list of numbers. In the body of for loop we are calculating the square of each number present in list and displaying the same.# Program to print squares of all numbers present in a list # List of integer numbers numbers = [1, 2, 4, 6, 11, 20] # variable to store the square of each num temporary sq = 0 # iterating over the given list for val in numbers: # calculating square of each number sq = val * val # displaying the squares print(sq)Output:
1 4 16 36 121 400
Function range()
In the above example, we have iterated over a list using for loop. However we can also use a range() function in for loop to iterate over numbers defined by range().range(n): generates a set of whole numbers starting from 0 to (n-1).
For example:
range(8) is equivalent to [0, 1, 2, 3, 4, 5, 6, 7]
range(start, stop): generates a set of whole numbers starting from
start
to stop-1
.For example:
range(5, 9) is equivalent to [5, 6, 7, 8]
range(start, stop, step_size): The default step_size is 1 which is why when we didn’t specify the step_size, the numbers generated are having difference of 1. However by specifying step_size we can generate numbers having the difference of step_size.
For example:
range(1, 10, 2) is equivalent to [1, 3, 5, 7, 9]
Lets use the range() function in for loop:
Python for loop example using range() function
Here we are using range() function to calculate and display the sum of first 5 natural numbers.# Program to print the sum of first 5 natural numbers # variable to store the sum sum = 0 # iterating over natural numbers using range() for val in range(1, 6): # calculating sum sum = sum + val # displaying sum of first 5 natural numbers print(sum)Output:
15
For loop with else block
Unlike Java, In Python we can have an optional ‘else’ block associated with the loop. The ‘else’ block executes only when the loop has completed all the iterations. Lets take an example:for val in range(5): print(val) else: print("The loop has completed execution")Output:
0 1 2 3 4 The loop has completed executionNote: The else block only executes when the loop is finished.
Nested For loop in Python
When a for loop is present inside another for loop then it is called a nested for loop. Lets take an example of nested for loop.for num1 in range(3): for num2 in range(10, 14): print(num1, ",", num2)Output:
0 , 10 0 , 11 0 , 12 0 , 13 1 , 10 1 , 11 1 , 12 1 , 13 2 , 10 2 , 11 2 , 12 2 , 13
Python While Loop
While loop is used to iterate over a block of code repeatedly until a given condition returns false. In the last tutorial, we have seen for loop in Python, which is also used for the same purpose. The main difference is that we use while loop when we are not
certain of the number of times the loop requires execution, on the
other hand when we exactly know how many times we need to run the loop,
we use for loop.Syntax of while loop
while condition: #body_of_whileThe body_of_while is set of Python statements which requires repeated execution. These set of statements execute repeatedly until the given condition returns false.
Flow of while loop
1. First the given condition is checked, if the condition returns false, the loop is terminated and the control jumps to the next statement in the program after the loop.2. If the condition returns true, the set of statements inside loop are executed and then the control jumps to the beginning of the loop for next iteration.
These two steps happen repeatedly as long as the condition specified in while loop remains true.
Python – While loop example
Here is an example of while loop. In this example, we have a variablenum
and we are displaying the value of num
in a loop, the loop has a increment operation where we are increasing the value of num
.
This is very important step, the while loop must have a increment or
decrement operation, else the loop will run indefinitely, we will cover
this later in infinite while loop.num = 1 # loop will repeat itself as long as # num < 10 remains true while num < 10: print(num) #incrementing the value of num num = num + 3Output:
1 4 7
Infinite while loop
Example 1:This will print the word ‘hello’ indefinitely because the condition will always be true.
while True: print("hello")Example 2:
num = 1 while num<5: print(num)This will print ‘1’ indefinitely because inside loop we are not updating the value of num, so the value of num will always remain 1 and the condition num < 5 will always return true.
Nested while loop in Python
When a while loop is present inside another while loop then it is called nested while loop. Lets take an example to understand this concept.i = 1 j = 5 while i < 4: while j < 8: print(i, ",", j) j = j + 1 i = i + 1Output:
1 , 5 2 , 6 3 , 7
Python – while loop with else block
We can have a ‘else’ block associated with while loop. The ‘else’ block is optional. It executes only after the loop finished execution.num = 10 while num > 6: print(num) num = num-1 else: print("loop is finished")Output:
10 9 8 7 loop is finished
Python break Statement
The break statement is used to terminate the loop when a certain condition is met. We already learned in previous tutorials (for loop and while loop) that a loop is used to iterate a set of statements repeatedly as long as the loop condition returns true. The break statement is generally used inside a loop along with a if statement so that when a particular condition (defined in if statement) returns true, the break statement is encountered and the loop terminates.For example, lets say we are searching an element in a list, so for that we are running a loop starting from the first element of the list to the last element of the list. Using break statement, we can terminate the loop as soon as the element is found because why run the loop unnecessary till the end of list when our element is found. We can achieve this with the help of break statement (we will see this example programmatically in the example section below).
Syntax of break statement in Python
The syntax of break statement in Python is similar to what we have seen in Java.break
Flow diagram of break
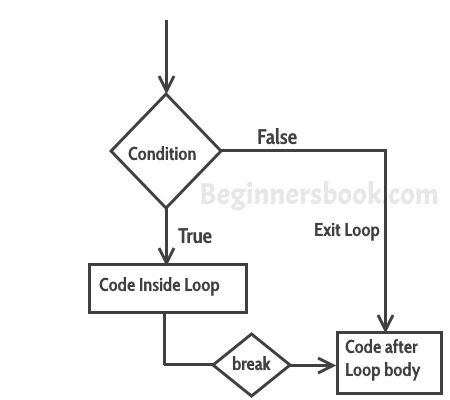
Example of break statement
In this example, we are searching a number ’88’ in the given list of numbers. The requirement is to display all the numbers till the number ’88’ is found and when it is found, terminate the loop and do not display the rest of the numbers.# program to display all the elements before number 88 for num in [11, 9, 88, 10, 90, 3, 19]: print(num) if(num==88): print("The number 88 is found") print("Terminating the loop") breakOutput:
11 9 88 The number 88 is found Terminating the loop
Note: You would always want to use the break statement with a if statement so that only when the condition associated with ‘if’ is true then only break is encountered. If you do not use it with ‘if’ statement then the break statement would be encountered in the first iteration of loop and the loop would always terminate on the first iteration.
Python Continue Statement
The continue statement is used inside a loop to skip the rest of the statements in the body of loop for the current iteration and jump to the beginning of the loop for next iteration. The break and continue statements are used to alter the flow of loop, break terminates the loop when a condition is met and continue skip the current iteration.Syntax of continue statement in Python
The syntax of continue statement in Python is similar to what we have seen in Java(except the semicolon)continue
Flow diagram of continue
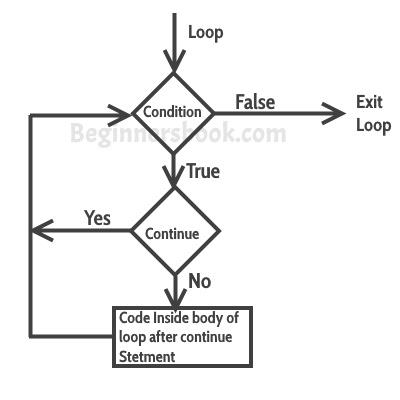
Example of continue statement
Lets say we have a list of numbers and we want to print only the odd numbers out of that list. We can do this by using continue statement.We are skipping the print statement inside loop by using continue statement when the number is even, this way all the even numbers are skipped and the print statement executed for all the odd numbers.
# program to display only odd numbers for num in [20, 11, 9, 66, 4, 89, 44]: # Skipping the iteration when number is even if num%2 == 0: continue # This statement will be skipped for all even numbers print(num)Output:
11 9 89
Python pass Statement
The pass statement acts as a placeholder and usually used when there is no need of code but a statement is still required to make a code syntactically correct. For example we want to declare a function in our code but we want to implement that function in future, which means we are not yet ready to write the body of the function. In this case we cannot leave the body of function empty as this would raise error because it is syntactically incorrect, in such cases we can use pass statement which does nothing but makes the code syntactically correct.Pass statement vs comment
You may be wondering that a python comment works similar to the pass statement as it does nothing so we can use comment in place of pass statement. Well, it is not the case, a comment is not a placeholder and it is completely ignored by the Python interpreter while on the other handpass
is not ignored by interpreter, it says the interpreter to do nothing.Python pass statement example
If the number is even we are doing nothing and if it is odd then we are displaying the number.for num in [20, 11, 9, 66, 4, 89, 44]: if num%2 == 0: pass else: print(num)Output:
11 9 89
Other examples:
A function that does nothing(yet), may be implemented in future.def f(arg): pass # a function that does nothing (yet)A class that does not have any methods(yet), may have methods in future implementation.
class C: pass # a class with no methods (yet)
Python Functions
we will learn about functions in Python. A function is a block of code that contains one or more Python statements and used for performing a specific task.
Why use function in Python?
As I mentioned above, a function is a block of code that performs a specific task. Lets discuss what we can achieve in Python by using functions in our code:1. Code re-usability: Lets say we are writing an application in Python where we need to perform a specific task in several places of our code, assume that we need to write 10 lines of code to do that specific task. It would be better to write those 10 lines of code in a function and just call the function wherever needed, because writing those 10 lines every time you perform that task is tedious, it would make your code lengthy, less-readable and increase the chances of human errors.
2. Improves Readability: By using functions for frequent tasks you make your code structured and readable. It would be easier for anyone to look at the code and be able to understand the flow and purpose of the code.
3. Avoid redundancy: When you no longer repeat the same lines of code throughout the code and use functions in places of those, you actually avoiding the redundancy that you may have created by not using functions.
Syntax of functions in Python
Function declaration:def function_name(function_parameters): function_body # Set of Python statements return # optional return statement Calling the function:
# when function doesn't return anything function_name(parameters) OR
# when function returns something # variable is to store the returned value variable = function_name(parameters)
Python Function example
Here we have a functionadd()
that adds two numbers
passed to it as parameters. Later after function declaration we are
calling the function twice in our program to perform the addition.def add(num1, num2): return num1 + num2 sum1 = add(100, 200) sum2 = add(8, 9) print(sum1) print(sum2) Output:
300 17
Default arguments in Function
Now that we know how to declare and call a function, lets see how can we use the default arguments. By using default arguments we can avoid the errors that may arise while calling a function without passing all the parameters. Lets take an example to understand this:In this example we have provided the default argument for the second parameter, this default argument would be used when we do not provide the second parameter while calling this function.
# default argument for second parameter def add(num1, num2=1): return num1 + num2 sum1 = add(100, 200) sum2 = add(8) # used default argument for second param sum3 = add(100) # used default argument for second param print(sum1) print(sum2) print(sum3) Output:
300 9 101
Types of functions
There are two types of functions in Python:1. Built-in functions: These functions are predefined in Python and we need not to declare these functions before calling them. We can freely invoke them as and when needed.
2. User defined functions: The functions which we create in our code are user-defined functions. The add() function that we have created in above examples is a user-defined function.
Python Recursion
A function is said to be a recursive if it calls itself. For example, lets say we have a functionabc()
and in the body of abc()
there is a call to the abc()
.Python example of Recursion
In this example we are defining a user-defined functionfactorial()
. This function finds the factorial of a number by calling itself repeatedly until the base case(We will discuss more about base case later, after this example) is reached.# Example of recursion in Python to # find the factorial of a given number def factorial(num): """This function calls itself to find the factorial of a number""" if num == 1: return 1 else: return (num * factorial(num - 1)) num = 5 print("Factorial of", num, "is: ", factorial(num))Output:
Factorial of 5 is: 120Lets see what happens in the above example:
factorial(5) returns 5 * factorial(5-1) i.e. 5 * factorial(4) |__5*4*factorial(3) |__5*4*3*factorial(2) |__5*4*3*2*factorial(1)Note: factorial(1) is a base case for which we already know the value of factorial. The base case is defined in the body of function with this code:
if num == 1: return 1
What is a base case in recursion
When working with recursion, we should define a base case for which we already know the answer. In the above example we are finding factorial of an integer number and we already know that the factorial of 1 is 1 so this is our base case.Each successive recursive call to the function should bring it closer to the base case, which is exactly what we are doing in above example.
We use base case in recursive function so that the function stops calling itself when the base case is reached. Without the base case, the function would keep calling itself indefinitely.
Why use recursion in programming?
We use recursion to break a big problem in small problems and those small problems into further smaller problems and so on. At the end the solutions of all the smaller subproblems are collectively helps in finding the solution of the big main problem.Advantages of recursion
Recursion makes our program:1. Easier to write.
2. Readable – Code is easier to read and understand.
3. Reduce the lines of code – It takes less lines of code to solve a problem using recursion.
Disadvantages of recursion
1. Not all problems can be solved using recursion.2. If you don’t define the base case then the code would run indefinitely.
3. Debugging is difficult in recursive functions as the function is calling itself in a loop and it is hard to understand which call is causing the issue.
4. Memory overhead – Call to the recursive function is not memory efficient.
Python Numbers
we will see how to work with numbers in Python. Python supports integers, floats and complex numbers.
An integer is a number without decimal point for example 5, 6, 10 etc.A float is a number with decimal point for example 6.7, 6.0, 10.99 etc.
A complex number has a real and imaginary part for example 7+8j, 8+11j etc.
Example: Numbers in Python
# Python program to display numbers of # different data types # int num1 = 10 num2 = 100 print(num1+num2) # float a = 10.5 b = 8.9 print(a-b) # complex numbers x = 3 + 4j y = 9 + 8j print(y-x)Output:
110 1.5999999999999996 (6+4j)
Python example to find the class(data type) of a number
We can use thetype()
function to find out the class of a number. An integer number belongs to int
class, a float number belongs to float
class and a complex number belongs to complex
class.# program to find the class of a number # int num = 100 print("type of num: ",type(num)) # float num2 = 10.99 print("type of num2: ",type(num2)) # complex numbers num3 = 3 + 4j print("type of num3: ",type(num3))Output:
The isinstance() function
The isinstance() functions checks whether a number belongs to a particular class and returns true or false based on the result.For example:
isinstance(num, int)
will return true if the number num
is an integer number.isinstance(num, int)
will return false if the number num
is not an integer number.Example of isinstance() function
num = 100 # true because num is an integer print(isinstance(num, int)) # false because num is not a float print(isinstance(num, float)) # false because num is not a complex number print(isinstance(num, complex))Output:
True False False
Python List with examples
we will discuss lists in Python. A list is a data
type that allows you to store various types data in it. List is a
compound data type which means you can have different-2 data types under
a list, for example we can have integer, float and string items in a
same list.
Create a List in Python
Lets see how to create a list in Python. To create a list all you have to do is to place the items inside a square bracket[]
separated by comma ,
.# list of floats num_list = [11.22, 9.9, 78.34, 12.0] # list of int, float and strings mix_list = [1.13, 2, 5, "beginnersbook", 100, "hi"] # an empty list nodata_list = []As we have seen above, a list can have data items of same type or different types. This is the reason list comes under compound data type.
Accessing the items of a list
Syntax to access the list items:list_name[index]Example:
# a list of numbers numbers = [11, 22, 33, 100, 200, 300] # prints 11 print(numbers[0]) # prints 300 print(numbers[5]) # prints 22 print(numbers[1])Output:
11 300 22Points to Note:
1. The index cannot be a float number.
For example:
# a list of numbers numbers = [11, 22, 33, 100, 200, 300] # error print(numbers[1.0])Output:
TypeError: list indices must be integers or slices, not float2. The index must be in range to avoid
IndexError
. The
range of the index of a list having 10 elements is 0 to 9, if we go
beyond 9 then we will get IndexError. However if we go below 0 then it
would not cause issue in certain cases, we will discuss that in our next
section.For example:
# a list of numbers numbers = [11, 22, 33, 100, 200, 300] # error print(numbers[6])Output:
IndexError: list index out of range
Negative Index to access the list items from the end
Unlike other programming languages where negative index may cause issue, Python allows you to use negative indexes. The idea behind this to allow you to access the list elements starting from the end. For example an index of-1
would access the last element of the list, -2
second last, -3
third last and so on.1 Example of Negative indexes in Python
# a list of strings my_list = ["hello", "world", "hi", "bye"] # prints "bye" print(my_list[-1]) # prints "world" print(my_list[-3]) # prints "hello" print(my_list[-4])Output:
bye
world
hello
How to get a sublist in Python using slicing
We can get a sublist from a list in Python using slicing operation. Lets say we have a listn_list
having 10 elements, then we can slice this list using colon :
operator. Lets take an example to understand this:1 Slicing example
# list of numbers n_list = [1, 2, 3, 4, 5, 6, 7] # list items from 2nd to 3rd print(n_list[1:3]) # list items from beginning to 3rd print(n_list[:3]) # list items from 4th to end of list print(n_list[3:]) # Whole list print(n_list[:])Output:
[2, 3] [1, 2, 3] [4, 5, 6, 7] [1, 2, 3, 4, 5, 6, 7]
List Operations
There are various operations that we can perform on Lists.1 Addition
There are several ways you can add elements to a list.# list of numbers n_list = [1, 2, 3, 4] # 1. adding item at the desired location # adding element 100 at the fourth location n_list.insert(3, 100) # list: [1, 2, 3, 100, 4] print(n_list) # 2. adding element at the end of the list n_list.append(99) # list: [1, 2, 3, 100, 4, 99] print(n_list) # 3. adding several elements at the end of list # the following statement can also be written like this: # n_list + [11, 22] n_list.extend([11, 22]) # list: [1, 2, 3, 100, 4, 99, 11, 22] print(n_list)Output:
[1, 2, 3, 100, 4] [1, 2, 3, 100, 4, 99] [1, 2, 3, 100, 4, 99, 11, 22]
2 Update elements
We can change the values of elements in a List. Lets take an example to understand this:# list of numbers n_list = [1, 2, 3, 4] # Changing the value of 3rd item n_list[2] = 100 # list: [1, 2, 100, 4] print(n_list) # Changing the values of 2nd to fourth items n_list[1:4] = [11, 22, 33] # list: [1, 11, 22, 33] print(n_list)Output:
[1, 2, 100, 4] [1, 11, 22, 33]
3 Delete elements
# list of numbers n_list = [1, 2, 3, 4, 5, 6] # Deleting 2nd element del n_list[1] # list: [1, 3, 4, 5, 6] print(n_list) # Deleting elements from 3rd to 4th del n_list[2:4] # list: [1, 3, 6] print(n_list) # Deleting the whole list del n_listOutput:
[1, 3, 4, 5, 6] [1, 3, 6]
4 Deleting elements using remove(), pop() and clear() methods
remove(item)
: Removes specified item from list.pop(index)
: Removes the element from the given index.pop()
: Removes the last element.clear()
: Removes all the elements from the list.# list of chars ch_list = ['A', 'F', 'B', 'Z', 'O', 'L'] # Deleting the element with value 'B' ch_list.remove('B') # list: ['A', 'F', 'Z', 'O', 'L'] print(ch_list) # Deleting 2nd element ch_list.pop(1) # list: ['A', 'Z', 'O', 'L'] print(ch_list) # Deleting all the elements ch_list.clear() # list: [] print(ch_list)Output:
['A', 'F', 'Z', 'O', 'L'] ['A', 'Z', 'O', 'L'] []
Python Strings
A string is usually a bit of text (sequence of characters). In Python we use ” (double quotes) or ‘ (single quotes) to represent a string. In this guide we will see how to create, access, use and manipulate strings in Python programming language.How to create a String in Python
There are several ways to create strings in Python.1. We can use ‘ (single quotes), see the string
str
in the following code.2. We can use ” (double quotes), see the string
str2
in the source code below.3. Triple double quotes “”” and triple single quotes ”’ are used for creating multi-line strings in Python. See the strings str3 and str4 in the following example.
# lets see the ways to create strings in Python str = 'beginnersbook' print(str) str2 = "Chaitanya" print(str2) # multi-line string str3 = """Welcome to Beginnersbook.com""" print(str3) str4 = '''This is a tech blog''' print(str4)Output:
beginnersbook Chaitanya Welcome to Beginnersbook.com This is a tech blog
How to access strings in Python
A string is nothing but an array of characters so we can use the indexes to access the characters of a it. Just like arrays, the indexes start from 0 to the length-1.You will get IndexError if you try to access the character which is not in the range. For example,
if a string is of length 6 and you try to access the 8th char of it then you will get this error.
You will get TypeError if you do not use integers as indexes, for example if you use a float as an index then you will get this error.
str = "Kevin" # displaying whole string print(str) # displaying first character of string print(str[0]) # displaying third character of string print(str[2]) # displaying the last character of the string print(str[-1]) # displaying the second last char of string print(str[-2])Output:
Kevin K v n i
Python String Operations
Lets see the operations that can be performed on the strings.1. Getting a substring in Python – Slicing operation
We can slice a string to get a substring out of it. To understand the concept of slicing we must understand the positive and negative indexes in Python (see the example above to understand this). Lets take a look at the few examples of slicing.str = "Beginnersbook" # displaying whole string print("The original string is: ", str) # slicing 10th to the last character print("str[9:]: ", str[9:]) # slicing 3rd to 6th character print("str[2:6]: ", str[2:6]) # slicing from start to the 9th character print("str[:9]: ", str[:9]) # slicing from 10th to second last character print("str[9:-1]: ", str[9:-1])Output:
The original string is: Beginnersbook str[9:]: book str[2:6]: ginn str[:9]: Beginners str[9:-1]: boo
2 Concatenation of strings in Python
The + operator is used for string concatenation in Python. Lets take an example to understand this:str1 = "One" str2 = "Two" str3 = "Three" # Concatenation of three strings print(str1 + str2 + str3)Output:
OneTwoThree
Note: When + operator is used on numbers it adds
them but when it used on strings it concatenates them. However if you
try to use this between string and number then it will throw TypeError.For example:
s = "one" n = 2 print(s+n)Output:
TypeError: must be str, not int
3 Repetition of string – Replication operator
We can use * operator to repeat a string by specified number of times.str = "ABC" # repeating the string str by 3 times print(str*3)Output:
ABCABCABC
4 Python Membership Operators in Strings
in: This checks whether a string is present in another string or not. It returns true if the entire string is found else it returns false.not in: It works just opposite to what “in” operator does. It returns true if the string is not found in the specified string else it returns false.
str = "Welcome to beginnersbook.com" str2 = "Welcome" str3 = "Chaitanya" str4 = "XYZ" # str2 is in str? True print(str2 in str) # str3 is in str? False print(str3 in str) # str4 not in str? True print(str4 not in str)Output:
True False True
5 Python – Relational Operators on Strings
The relational operators works on strings based on the ASCII values of characters.The ASCII value of a is 97, b is 98 and so on.
The ASCII value of A is 65, B is 66 and so on.
str = "ABC" str2 = "aBC" str3 = "XYZ" str4 = "XYz" # ASCII value of str2 is > str? True print(str2 > str) # ASCII value of str3 is > str4? False print(str3 > str4)Output:
True False
Python Tuple with example
a tuple is similar to List except that the objects in tuple are immutable which means we cannot change the elements of a tuple once assigned. On the other hand, we can change the elements of a list.
Tuple vs List
1. The elements of a list are mutable whereas the elements of a tuple are immutable.2. When we do not want to change the data over time, the tuple is a preferred data type whereas when we need to change the data in future, list would be a wise option.
3. Iterating over the elements of a tuple is faster compared to iterating over a list.
4. Elements of a tuple are enclosed in parenthesis whereas the elements of list are enclosed in square bracket.
How to create a tuple in Python
To create a tuple in Python, place all the elements in a () parenthesis, separated by commas. A tuple can have heterogeneous data items, a tuple can have string and list as data items as well.1 Example – Creating tuple
In this example, we are creating few tuples. We can have tuple of same type of data items as well as mixed type of data items. This example also shows nested tuple (tuples as data items in another tuple).# tuple of strings my_data = ("hi", "hello", "bye") print(my_data) # tuple of int, float, string my_data2 = (1, 2.8, "Hello World") print(my_data2) # tuple of string and list my_data3 = ("Book", [1, 2, 3]) print(my_data3) # tuples inside another tuple # nested tuple my_data4 = ((2, 3, 4), (1, 2, "hi")) print(my_data4)Output:
('hi', 'hello', 'bye') (1, 2.8, 'Hello World') ('Book', [1, 2, 3]) ((2, 3, 4), (1, 2, 'hi'))
2 Empty tuple:
# empty tuple my_data = ()
3 Tuple with only single element:
Note: When a tuple has only one element, we must put a comma after the element, otherwise Python will not treat it as a tuple.# a tuple with single data item my_data = (99,)
If we do not put comma after 99 in the above example then python will treat my_data as an int variable rather than a tuple.
How to access tuple elements
We use indexes to access the elements of a tuple. Lets take few example to understand the working.1 Accessing tuple elements using positive indexes
We can also have negative indexes in tuple, we have discussed that in the next section. Indexes starts with 0 that is why we use 0 to access the first element of tuple, 1 to access second element and so on.# tuple of strings my_data = ("hi", "hello", "bye") # displaying all elements print(my_data) # accessing first element # prints "hi" print(my_data[0]) # accessing third element # prints "bye" print(my_data[2])Output:
('hi', 'hello', 'bye') hi byeNote:
1. TypeError: If you do not use integer indexes in the tuple. For example my_data[2.0] will raise this error. The index must always be an integer.
2. IndexError: Index out of range. This error occurs when we mention the index which is not in the range. For example, if a tuple has 5 elements and we try to access the 7th element then this error would occurr.
2 Negative indexes in tuples
Similar to list and strings we can use negative indexes to access the tuple elements from the end.-1 to access last element, -2 to access second last and so on.
my_data = (1, 2, "Kevin", 8.9) # accessing last element # prints 8.9 print(my_data[-1]) # prints 2 print(my_data[-3])Output:
8.9 2
3 Accessing elements from nested tuples
Lets understand how the double indexes are used to access the elements of nested tuple. The first index represents the element of main tuple and the second index represent the element of the nested tuple.In the following example, when I used my_data[2][1], it accessed the second element of the nested tuple. Because 2 represented the third element of main tuple which is a tuple and the 1 represented the second element of that tuple.
my_data = (1, "Steve", (11, 22, 33)) # prints 'v' print(my_data[1][3]) # prints 22 print(my_data[2][1])Output:
v 22
Operations that can be performed on tuple in Python
Lets see the operations that can be performed on the tuples in Python.1 Changing the elements of a tuple
We cannot change the elements of a tuple because elements of tuple are immutable. However we can change the elements of nested items that are mutable. For example, in the following code, we are changing the element of the list which is present inside the tuple. List items are mutable that’s why it is allowed.my_data = (1, [9, 8, 7], "World") print(my_data) # changing the element of the list # this is valid because list is mutable my_data[1][2] = 99 print(my_data) # changing the element of tuple # This is not valid since tuple elements are immutable # TypeError: 'tuple' object does not support item assignment # my_data[0] = 101 # print(my_data)Output:
(1, [9, 8, 7], 'World') (1, [9, 8, 99], 'World')
2 Delete operation on tuple
We already discussed above that tuple elements are immutable which also means that we cannot delete the elements of a tuple. However deleting entire tuple is possible.my_data = (1, 2, 3, 4, 5, 6) print(my_data) # not possible # error # del my_data[2] # deleting entire tuple is possible del my_data # not possible # error # because my_data is deleted # print(my_data)Output:
(1, 2, 3, 4, 5, 6)
3 Slicing operation in tuples
my_data = (11, 22, 33, 44, 55, 66, 77, 88, 99) print(my_data) # elements from 3rd to 5th # prints (33, 44, 55) print(my_data[2:5]) # elements from start to 4th # prints (11, 22, 33, 44) print(my_data[:4]) # elements from 5th to end # prints (55, 66, 77, 88, 99) print(my_data[4:]) # elements from 5th to second last # prints (55, 66, 77, 88) print(my_data[4:-1]) # displaying entire tuple print(my_data[:])Output:
(11, 22, 33, 44, 55, 66, 77, 88, 99) (33, 44, 55) (11, 22, 33, 44) (55, 66, 77, 88, 99) (55, 66, 77, 88) (11, 22, 33, 44, 55, 66, 77, 88, 99)
4 Membership Test in Tuples
in: Checks whether an element exists in the specified tuple.not in: Checks whether an element does not exist in the specified tuple.
my_data = (11, 22, 33, 44, 55, 66, 77, 88, 99) print(my_data) # true print(22 in my_data) # false print(2 in my_data) # false print(88 not in my_data) # true print(101 not in my_data)Output:
(11, 22, 33, 44, 55, 66, 77, 88, 99) True False False True
5 Iterating a tuple
# tuple of fruits my_tuple = ("Apple", "Orange", "Grapes", "Banana") # iterating over tuple elements for fruit in my_tuple: print(fruit)Output:
Apple Orange Grapes Banana
Python Dictionary with examples
Dictionary is a mutable data type in Python. A python dictionary is a
collection of key and value pairs separated by a colon (:), enclosed in
curly braces {}.
Python Dictionary
Here we have a dictionary. Left side of the colon(:) is the key and right side of the : is the value.mydict = {'StuName': 'Ajeet', 'StuAge': 30, 'StuCity': 'Agra'}Points to Note:
1. Keys must be unique in dictionary, duplicate values are allowed.
2. A dictionary is said to be empty if it has no key value pairs. An empty dictionary is denoted like this: {}.
3. The keys of dictionary must be of immutable data types such as String, numbers or tuples.
Accessing dictionary values using keys in Python
To access a value we can can use the corresponding key in the square brackets as shown in the following example. Dictionary name followed by square brackets and in the brackets we specify the key for which we want the value.mydict = {'StuName': 'Ajeet', 'StuAge': 30, 'StuCity': 'Agra'} print("Student Age is:", mydict['StuAge']) print("Student City is:", mydict['StuCity'])Output:
If you specify a key which doesn’t exist in the dictionary then you will get a compilation error. For example. Here we are trying to access the value for key ‘StuClass’ which does not exist in the dictionary
mydict
, thus we get a compilation error when we run this code.mydict = {'StuName': 'Ajeet', 'StuAge': 30, 'StuCity': 'Agra'} print("Student Age is:", mydict['StuClass']) print("Student City is:", mydict['StuCity'])Output:
Change values in Dictionary
Here we are updating the values for the existing key-value pairs. To update a value in dictionary we are using the corresponding key.mydict = {'StuName': 'Ajeet', 'StuAge': 30, 'StuCity': 'Agra'} print("Student Age before update is:", mydict['StuAge']) print("Student City before update is:", mydict['StuCity']) mydict['StuAge'] = 31 mydict['StuCity'] = 'Noida' print("Student Age after update is:", mydict['StuAge']) print("Student City after update is:", mydict['StuCity'])Output:
Adding a new entry (key-value pair) in dictionary
We can also add a new key-value pair in an existing dictionary. Lets take an example to understand this.mydict = {'StuName': 'Steve', 'StuAge': 4, 'StuCity': 'Agra'} mydict['StuClass'] = 'Jr.KG' print("Student Name is:", mydict['StuName']) print("Student Class is:", mydict['StuClass'])Output:
Loop through a dictionary
We can loop through a dictionary as shown in the following example. Here we are using for loop.mydict = {'StuName': 'Steve', 'StuAge': 4, 'StuCity': 'Agra'} for e in mydict: print("Key:",e,"Value:",mydict[e])Output:
Python delete operation on dictionary
We can delete key-value pairs as well as entire dictionary in python. Lets take an example. As you can see we can use del following by dictionary name and in square brackets we can specify the key to delete the specified key value pair from dictionary.To delete all the entries (all key-value pairs) from dictionary we can use the clear() method.
To delete entire dictionary along with all the data use del keyword followed by dictionary name as shown in the following example.
mydict = {'StuName': 'Steve', 'StuAge': 4, 'StuCity': 'Agra'} del mydict['StuCity']; # remove entry with key 'StuCity' mydict.clear(); # remove all key-value pairs from mydict del mydict ; # delete entire dictionary mydict
Python Sets
Set is an unordered and unindexed collection of items in Python.
Unordered means when we display the elements of a set, it will come out
in a random order. Unindexed means, we cannot access the elements of a
set using the indexes like we can do in list and tuples.
The elements of a set are defined inside square brackets and are separated by commas. For example –myset = [1, 2, 3, 4, "hello"]
Python Set Example
# Set Example myset = {"hi", 2, "bye", "Hello World"} print(myset)Output:
Checking whether an item is in the set
We can check whether an item exists in Set or not using “in” operator as shown in the following example. This returns the boolean value true or false. If the item is in the given set then it returns true, else it returns false.# Set Example myset = {"hi", 2, "bye", "Hello World"} # checking whether 2 is in myset print(2 in myset) # checking whether "hi" is in myset print("hi" in myset) # checking whether "BeginnersBook" is in myset print("BeginnersBook" in myset)Output:
Loop through the elements of a Set in Python
We can loop through the elements of a set in Python as shown in the following elements. As you can see in the output that the elements will appear in random order each time you run the code.# Set Example myset = {"hi", 2, "bye", "Hello World"} # loop through the elements of myset for a in myset: print(a)Output:
Python – Add or remove item from a Set
We can add an item in a Set using add() function and we can remove an item from a set using remove() function as shown in the following example.# Set Example myset = {"hi", 2, "bye", "Hello World"} print("Original Set:", myset) # adding an item myset.add(99) print("Set after adding 99:", myset) # removing an item myset.remove("bye") print("Set after removing bye:", myset)Output:
Set Methods
1. add(): This method adds an element to the Set.2. remove(): This method removes a specified element from the Set
3. discard(): This method works same as remove() method, however it doesn’t raise an error when the specified element doesn’t exist.
4. clear(): Removes all the elements from the set.
5. copy(): Returns a shallow copy of the set.
6. difference(): This method returns a new set which is a difference between two given sets.
7. difference_update(): Updates the calling set with the Set difference of two given sets.
8. intersection(): Returns a new set which contains the elements that are common to all the sets.
9. intersection_update(): Updates the calling set with the Set intersection of two given sets.
10. isdisjoint(): Checks whether two sets are disjoint or not. Two sets are disjoint if they have no common elements.
11. issubset(): Checks whether a set is a subset of another given set.
12. pop(): Removes and returns a random element from the set.
13. union(): Returns a new set with the distinct elements of all the sets.
14. update(): Adds elements to a set from other passed iterable.
15. symmetric_difference(): Returns a new set which is a symmetric difference of two given sets.
16. symmetric_difference_update(): Updates the calling set with the symmetric difference of two given sets.
Python OOPs Concepts
Python is an object-oriented programming language. What this means is we can solve a problem in Python by creating objects in our programs. In this guide, we will discuss OOPs terms such as class, objects, methods etc. along with the Object oriented programming features such as inheritance, polymorphism, abstraction, encapsulation.Object
An object is an entity that has attributes and behaviour. For example,Ram
is an object who has attributes such as height, weight, color etc. and
has certain behaviours such as walking, talking, eating etc.Class
A class is a blueprint for the objects. For example, Ram, Shyam, Steve, Rick are all objects so we can define a template (blueprint) classHuman
for these objects. The class can define the common attributes and behaviours of all the objects.Methods
As we discussed above, an object has attributes and behaviours. These behaviours are called methods in programming.Example of Class and Objects
In this example, we have two objectsRam
and Steve
that belong to the class Human
Object attributes: name, height, weight
Object behaviour: eating()
How to create Class and Objects in Python
we will see how to create classes and objects in Python.
Define class in Python
A class is defined using the keywordclass
.Example
In this example, we are creating an empty classDemoClass
. This class has no attributes and methods.The string that we mention in the triple quotes is a docstring which is an optional string that briefly explains the purpose of the class.
class DemoClass: """This is my docstring, this explains brief about the class""" # this prints the docstring of the class print(DemoClass.__doc__)Output:
This is my docstring, this explains brief about the class
Creating Objects of class
In this example, we have a classMyNewClass
that has an attribute num
and a function hello()
. We are creating an object obj
of the class and accessing the attribute value of object and calling the method hello() using the object.class MyNewClass: """This class demonstrates the creation of objects""" # instance attribute num = 100 # instance method def hello(self): print("Hello World!") # creating object of MyNewClass obj = MyNewClass() # prints attribute value print(obj.num) # calling method hello() obj.hello() # prints docstring print(MyNewClass.__doc__)Output:
100 Hello World! This class demonstrates the creation of objects
Python Constructors – default and parameterized
A constructor is a special kind of method which is used for initializing
the instance variables during object creation. In this guide, we will
see what is a constructor, types of it and how to use them in the python
programming with examples.
1. What is a Constructor in Python?
Constructor is used for initializing the instance members when we create the object of a class.For example:
Here we have a instance variable
num
which we are
initializing in the constructor. The constructor is being invoked when
we create the object of the class (obj in the following example).class DemoClass: # constructor def __init__(self): # initializing instance variable self.num=100 # a method def read_number(self): print(self.num) # creating object of the class. This invokes constructor obj = DemoClass() # calling the instance method using the object obj obj.read_number()Output:
100
1.1 Syntax of constructor declaration
As we have seen in the above example that a constructor always has a nameinit
and the name init is prefixed and suffixed with a double underscore(__). We declare a constructor using def
keyword, just like methods.def __init__(self): # body of the constructor
2. Types of constructors in Python
We have two types of constructors in Python.1. default constructor – this is the one, which we have seen in the above example. This constructor doesn’t accept any arguments.
2. parameterized constructor – constructor with parameters is known as parameterized constructor.
2.1 Python – default constructor example
Note: An object cannot be created if we don’t have a constructor in our program. This is why when we do not declare a constructor in our program, python does it for us. Lets have a look at the example below.Example: When we do not declare a constructor
In this example, we do not have a constructor but still we are able to create an object for the class. This is because there is a default constructor implicitly injected by python during program compilation, this is an empty default constructor that looks like this:
def __init__(self): # no body, does nothing.Source Code:
class DemoClass: num = 101 # a method def read_number(self): print(self.num) # creating object of the class obj = DemoClass() # calling the instance method using the object obj obj.read_number()Output:
101
Example: When we declare a constructorIn this case, python does not create a constructor in our program.
class DemoClass: num = 101 # non-parameterized constructor def __init__(self): self.num = 999 # a method def read_number(self): print(self.num) # creating object of the class obj = DemoClass() # calling the instance method using the object obj obj.read_number()Output:
999
2.2 Python – Parameterized constructor example
When we declare a constructor in such a way that it accepts the arguments during object creation then such type of constructors are known as Parameterized constructors. As you can see that with such type of constructors we can pass the values (data) during object creation, which is used by the constructor to initialize the instance members of that object.class DemoClass: num = 101 # parameterized constructor def __init__(self, data): self.num = data # a method def read_number(self): print(self.num) # creating object of the class # this will invoke parameterized constructor obj = DemoClass(55) # calling the instance method using the object obj obj.read_number() # creating another object of the class obj2 = DemoClass(66) # calling the instance method using the object obj obj2.read_number()Output:
55 66
Comments
Post a Comment